Oh noes!
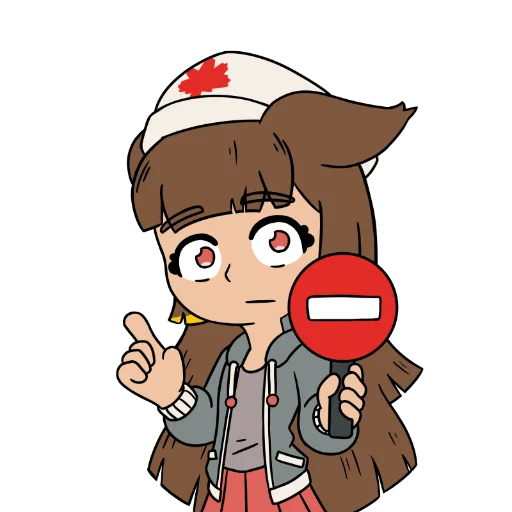
Access Denied: error code b21cf2fe29e8c885c3b73e821b48aea37e70fa76417c8125caac886ece3ce65f.
Go home or if you believe you should not be blocked, please contact the webmaster at iliana@buttslol.net
Access Denied: error code b21cf2fe29e8c885c3b73e821b48aea37e70fa76417c8125caac886ece3ce65f.
Go home or if you believe you should not be blocked, please contact the webmaster at iliana@buttslol.net